Class ListBox
A widget that presents a list of choices to the user, either as a list box or
as a drop-down list.
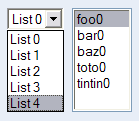
CSS Style Rules
Example
public class ListBoxExample implements EntryPoint {
public void onModuleLoad() {
// Make a new list box, adding a few items to it.
ListBox lb = new ListBox();
lb.addItem("foo");
lb.addItem("bar");
lb.addItem("baz");
lb.addItem("toto");
lb.addItem("tintin");
// Make enough room for all five items (setting this value to 1 turns it
// into a drop-down list).
lb.setVisibleItemCount(5);
// Add it to the root panel.
RootPanel.get().add(lb);
}
}
Constructors
Methods
Constructor Detail
ListBox
public ListBox()
Creates an empty list box in single selection mode.
ListBox
public ListBox(boolean isMultipleSelect)
Creates an empty list box. The preferred way to enable multiple selections
is to use this constructor rather than
setMultipleSelect(boolean).
Parameters
- isMultipleSelect
- specifies if multiple selection is enabled
Method Detail
addChangeListener
Adds a listener interface to receive change events.
Parameters
- listener
- the listener interface to add
addItem
public void
addItem(
String item)
Adds an item to the list box. This method has the same effect as
addItem(item, item)
Parameters
- item
- the text of the item to be added
addItem
Adds an item to the list box, specifying an initial value for the item.
Parameters
- item
- the text of the item to be added
- value
- the item's value, to be submitted if it is part of a
FormPanel; cannot be
null
clear
public void clear()
Removes all items from the list box.
getItemCount
public int getItemCount()
Gets the number of items present in the list box.
Return Value
the number of items
getItemText
public
String getItemText(
int index)
Gets the text associated with the item at the specified index.
Parameters
- index
- the index of the item whose text is to be retrieved
Return Value
the text associated with the item
getName
Gets the widget's name.
Return Value
the widget's name
getSelectedIndex
public int getSelectedIndex()
Gets the currently-selected item. If multiple items are selected, this
method will return the first selected item (
isItemSelected(int)
can be used to query individual items).
Return Value
the selected index, or
-1
if none is selected
getValue
public
String getValue(
int index)
Gets the value associated with the item at a given index.
Parameters
- index
- the index of the item to be retrieved
Return Value
the item's associated value
getVisibleItemCount
public int getVisibleItemCount()
Gets the number of items that are visible. If only one item is visible,
then the box will be displayed as a drop-down list.
Return Value
the visible item count
insertItem
public void
insertItem(
String item, int index)
Inserts an item into the list box. Has the same effect as
insertItem(item, item, index)
Parameters
- item
- the text of the item to be inserted
- index
- the index at which to insert it
insertItem
public void
insertItem(
String item, String value, int index)
Inserts an item into the list box, specifying an initial value for the
item. If the index is less than zero, or greater than or equal to
the length of the list, then the item will be appended to the end of
the list.
Parameters
- item
- the text of the item to be inserted
- value
- the item's value, to be submitted if it is part of a
FormPanel.
- index
- the index at which to insert it
isItemSelected
public boolean isItemSelected(int index)
Determines whether an individual list item is selected.
Parameters
- index
- the index of the item to be tested
Return Value
true
if the item is selected
isMultipleSelect
public boolean isMultipleSelect()
Gets whether this list allows multiple selection.
Return Value
true
if multiple selection is allowed
onBrowserEvent
public void
onBrowserEvent(
Event event)
Parameters
- event
-
removeChangeListener
Removes a previously added listener interface.
Parameters
- listener
- the listener interface to remove
removeItem
public void removeItem(int index)
Removes the item at the specified index.
Parameters
- index
- the index of the item to be removed
setItemSelected
public void setItemSelected(int index, boolean selected)
Sets whether an individual list item is selected.
Note that setting the selection programmatically does not cause
the ChangeListener.onChange(Widget) event to be fired.
Parameters
- index
- the index of the item to be selected or unselected
- selected
-
true
to select the item
setItemText
public void
setItemText(
int index, String text)
Sets the text associated with the item at a given index.
Parameters
- index
- the index of the item to be set
- text
- the item's new text
setMultipleSelect
public void setMultipleSelect(boolean multiple)
Sets whether this list allows multiple selections.
NOTE: The preferred
way of enabling multiple selections in a list box is by using the
ListBox(boolean) constructor. Using this method can spuriously
fail on Internet Explorer 6.0.
Parameters
- multiple
-
true
to allow multiple selections
setName
public void
setName(
String name)
Sets the widget's name.
Parameters
- name
- the widget's new name
setSelectedIndex
public void setSelectedIndex(int index)
Sets the currently selected index.
Note that setting the selected index programmatically does not
cause the ChangeListener.onChange(Widget) event to be fired.
Parameters
- index
- the index of the item to be selected
setValue
public void
setValue(
int index, String value)
Sets the value associated with the item at a given index. This value can be
used for any purpose, but is also what is passed to the server when the
list box is submitted as part of a
FormPanel.
Parameters
- index
- the index of the item to be set
- value
- the item's new value; cannot be
null
setVisibleItemCount
public void setVisibleItemCount(int visibleItems)
Sets the number of items that are visible. If only one item is visible,
then the box will be displayed as a drop-down list.
Parameters
- visibleItems
- the visible item count