Class RichTextArea
A rich text editor that allows complex styling and formatting.
Because some browsers do not support rich text editing, and others support
only a limited subset of functionality, there are two formatter interfaces,
accessed via
getBasicFormatter() and
getExtendedFormatter().
A browser that does not support rich text editing at all will return
null
for both of these, while one that supports only the basic
functionality will return
null
for the latter.
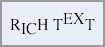
CSS Style Rules
Nested Classes
Nested Interfaces
Constructors
Methods
Constructor Detail
RichTextArea
public RichTextArea()
Creates a new, blank
RichTextArea object with no stylesheet.
Method Detail
addMouseListener
Adds a listener interface to receive mouse events.
Parameters
- listener
- the listener interface to add
getBasicFormatter
Gets the basic rich text formatting interface.
Return Value
null
if basic formatting is not supported
getExtendedFormatter
Gets the full rich text formatting interface.
Return Value
null
if full formatting is not supported
getHTML
Gets this object's contents as HTML.
Return Value
the object's HTML
getText
Gets this object's text.
Return Value
the object's text
onAttach
protected void onAttach()
This method is called when a widget is attached to the browser's document.
To receive notification after a Widget has been added to the document,
override the
onLoad method.
Subclasses that override this method must call
super.onAttach()
to ensure that the Widget has been attached
to its underlying Element.
onBrowserEvent
public void
onBrowserEvent(
Event event)
Parameters
- event
-
onDetach
protected void onDetach()
This method is called when a widget is detached from the browser's
document. To receive notification before a Widget is removed from the
document, override the
onUnload method.
Subclasses that override this method must call
super.onDetach()
to ensure that the Widget has been detached
from the underlying Element. Failure to do so will result in application
memory leaks due to circular references between DOM Elements and JavaScript
objects.
removeMouseListener
Removes a previously added listener interface.
Parameters
- listener
- the listener interface to remove
setFocus
public void setFocus(boolean focused)
Parameters
- focused
-
setHTML
public void
setHTML(
String html)
Sets this object's contents via HTML. Use care when setting an object's
HTML; it is an easy way to expose script-based security problems. Consider
using
setText(String) whenever possible.
Parameters
- html
- the object's new HTML
setText
public void
setText(
String text)
Sets this object's text.
Parameters
- text
- the object's new text