Class StackPanel
A panel that stacks its children vertically, displaying only one at a time,
with a header for each child which the user can click to display.
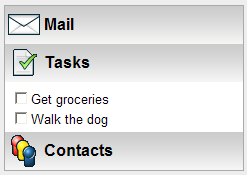
CSS Style Rules
- .gwt-StackPanel { the panel itself }
- .gwt-StackPanel .gwt-StackPanelItem { unselected items }
- .gwt-StackPanel .gwt-StackPanelItem-selected { selected items }
Example
public class StackPanelExample implements EntryPoint {
public void onModuleLoad() {
// Create a stack panel containing three labels.
StackPanel panel = new StackPanel();
panel.add(new Label("Foo"), "foo");
panel.add(new Label("Bar"), "bar");
panel.add(new Label("Baz"), "baz");
// Add it to the root panel.
RootPanel.get().add(panel);
}
}
Constructors
Methods
Constructor Detail
StackPanel
public StackPanel()
Creates an empty stack panel.
Method Detail
add
Adds a new child with the given widget.
Parameters
- w
- the widget to be added
add
Adds a new child with the given widget and header.
Parameters
- w
- the widget to be added
- stackText
- the header text associated with this widget
add
Adds a new child with the given widget and header, optionally interpreting
the header as HTML.
Parameters
- w
- the widget to be added
- stackText
- the header text associated with this widget
- asHTML
-
true
to treat the specified text as HTML
getSelectedIndex
public int getSelectedIndex()
Gets the currently selected child index.
Return Value
selected child
insert
public void
insert(
Widget w, int beforeIndex)
Inserts a widget before the specified index.
Parameters
- w
- the widget to be inserted
- beforeIndex
- the index before which it will be inserted
onBrowserEvent
public void
onBrowserEvent(
Event event)
Parameters
- event
-
remove
public boolean remove(int index)
Parameters
- index
-
remove
public boolean
remove(
Widget w)
Parameters
- w
-
setStackText
public void
setStackText(
int index, String text)
Sets the text associated with a child by its index.
Parameters
- index
- the index of the child whose text is to be set
- text
- the text to be associated with it
setStackText
public void
setStackText(
int index, String text, boolean asHTML)
Sets the text associated with a child by its index.
Parameters
- index
- the index of the child whose text is to be set
- text
- the text to be associated with it
- asHTML
-
true
to treat the specified text as HTML
showStack
public void showStack(int index)
Shows the widget at the specified child index.
Parameters
- index
- the index of the child to be shown