Class TabPanel
A panel that represents a tabbed set of pages, each of which contains another
widget. Its child widgets are shown as the user selects the various tabs
associated with them. The tabs can contain arbitrary HTML.
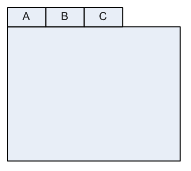
Note that this widget is not a panel per se, but rather a
Composite that aggregates a
TabBar and a
DeckPanel. It does, however, implement
HasWidgets.
CSS Style Rules
- .gwt-TabPanel { the tab panel itself }
- .gwt-TabPanelBottom { the bottom section of the tab panel (the deck
containing the widget) }
Example
public class TabPanelExample implements EntryPoint {
public void onModuleLoad() {
// Create a tab panel with three tabs, each of which displays a different
// piece of text.
TabPanel tp = new TabPanel();
tp.add(new HTML("Foo"), "foo");
tp.add(new HTML("Bar"), "bar");
tp.add(new HTML("Baz"), "baz");
// Show the 'bar' tab initially.
tp.selectTab(1);
// Add it to the root panel.
RootPanel.get().add(tp);
}
}
Constructors
Methods
add(Widget) | Adds a child widget. |
add(Widget, String) | Adds a widget to the tab panel. |
add(Widget, String, boolean) | Adds a widget to the tab panel. |
add(Widget, Widget) | Adds a widget to the tab panel. |
addTabListener(TabListener) | Adds a listener interface to receive click events. |
clear() | Removes all child widgets. |
getDeckPanel() | Gets the deck panel within this tab panel. |
getTabBar() | Gets the tab bar within this tab panel. |
getWidget(int) | Gets the child widget at the specified index. |
getWidgetCount() | Gets the number of child widgets in this panel. |
getWidgetIndex(Widget) | Gets the index of the specified child widget. |
insert(Widget, String, boolean, int) | Inserts a widget into the tab panel. |
insert(Widget, Widget, int) | Inserts a widget into the tab panel. |
insert(Widget, String, int) | Inserts a widget into the tab panel. |
iterator() | Gets an iterator for the contained widgets. |
onBeforeTabSelected(SourcesTabEvents, int) | Fired just before a tab is selected. |
onTabSelected(SourcesTabEvents, int) | Fired when a tab is selected. |
remove(int) | Removes the widget at the specified index. |
remove(Widget) | Removes the given widget, and its associated tab. |
removeTabListener(TabListener) | Removes a previously added listener interface. |
selectTab(int) | Programmatically selects the specified tab. |
Constructor Detail
TabPanel
public TabPanel()
Creates an empty tab panel.
Method Detail
add
Adds a child widget.
Parameters
- w
- the widget to be added
add
Adds a widget to the tab panel. If the Widget is already attached to the
TabPanel, it will be moved to the right-most index.
Parameters
- w
- the widget to be added
- tabText
- the text to be shown on its tab
add
Adds a widget to the tab panel. If the Widget is already attached to the
TabPanel, it will be moved to the right-most index.
Parameters
- w
- the widget to be added
- tabText
- the text to be shown on its tab
- asHTML
-
true
to treat the specified text as HTML
add
Adds a widget to the tab panel. If the Widget is already attached to the
TabPanel, it will be moved to the right-most index.
Parameters
- w
- the widget to be added
- tabWidget
- the widget to be shown in the tab
addTabListener
Adds a listener interface to receive click events.
Parameters
- listener
- the listener interface to add
clear
public void clear()
Removes all child widgets.
getDeckPanel
Gets the deck panel within this tab panel. Adding or removing Widgets from
the DeckPanel is not supported and will throw
UnsupportedOperationExceptions.
Return Value
the deck panel
getTabBar
Gets the tab bar within this tab panel. Adding or removing tabs from from
the TabBar is not supported and will throw UnsupportedOperationExceptions.
Return Value
the tab bar
getWidget
public
Widget getWidget(
int index)
Gets the child widget at the specified index.
Parameters
- index
- the child widget's index
Return Value
the child widget
getWidgetCount
public int getWidgetCount()
Gets the number of child widgets in this panel.
Return Value
the number of children
getWidgetIndex
public int
getWidgetIndex(
Widget child)
Gets the index of the specified child widget.
Parameters
- child
- the widget to be found
Return Value
the widget's index, or
-1
if it is not a child of
this panel
insert
public void
insert(
Widget widget, String tabText, boolean asHTML, int beforeIndex)
Inserts a widget into the tab panel. If the Widget is already attached to
the TabPanel, it will be moved to the requested index.
Parameters
- widget
- the widget to be inserted
- tabText
- the text to be shown on its tab
- asHTML
-
true
to treat the specified text as HTML
- beforeIndex
- the index before which it will be inserted
insert
public void
insert(
Widget widget, Widget tabWidget, int beforeIndex)
Inserts a widget into the tab panel. If the Widget is already attached to
the TabPanel, it will be moved to the requested index.
Parameters
- widget
- the widget to be inserted.
- tabWidget
- the widget to be shown on its tab.
- beforeIndex
- the index before which it will be inserted.
insert
public void
insert(
Widget widget, String tabText, int beforeIndex)
Inserts a widget into the tab panel. If the Widget is already attached to
the TabPanel, it will be moved to the requested index.
Parameters
- widget
- the widget to be inserted
- tabText
- the text to be shown on its tab
- beforeIndex
- the index before which it will be inserted
iterator
Gets an iterator for the contained widgets. This iterator is required to
implement
Iterator.remove().
onBeforeTabSelected
Fired just before a tab is selected.
Parameters
- sender
- the TabBar or TabPanel whose tab was
selected.
- tabIndex
- the index of the tab about to be selected
Return Value
false
to disallow the selection. If any listener
returns false, then the selection will be disallowed.
onTabSelected
Fired when a tab is selected.
Parameters
- sender
- the TabBar or TabPanel whose tab was selected
- tabIndex
- the index of the tab that was selected
remove
public boolean remove(int index)
Removes the widget at the specified index.
Parameters
- index
- the index of the widget to be removed
Return Value
false
if the widget is not present
remove
public boolean
remove(
Widget widget)
Removes the given widget, and its associated tab.
Parameters
- widget
- the widget to be removed
removeTabListener
Removes a previously added listener interface.
Parameters
- listener
- the listener interface to remove
selectTab
public void selectTab(int index)
Programmatically selects the specified tab.
Parameters
- index
- the index of the tab to be selected