Class FlexTable
A flexible table that creates cells on demand. It can be jagged (that is,
each row can contain a different number of cells) and individual cells can be
set to span multiple rows or columns.
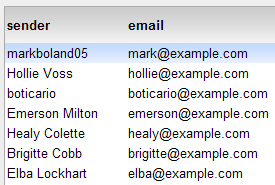
Example
public class FlexTableExample implements EntryPoint {
public void onModuleLoad() {
// Tables have no explicit size -- they resize automatically on demand.
FlexTable t = new FlexTable();
// Put some text at the table's extremes. This forces the table to be
// 3 by 3.
t.setText(0, 0, "upper-left corner");
t.setText(2, 2, "bottom-right corner");
// Let's put a button in the middle...
t.setWidget(1, 0, new Button("Wide Button"));
// ...and set it's column span so that it takes up the whole row.
t.getFlexCellFormatter().setColSpan(1, 0, 3);
RootPanel.get().add(t);
}
}
Nested Classes
Constructors
Methods
Constructor Detail
FlexTable
public FlexTable()
Method Detail
addCell
public void addCell(int row)
Appends a cell to the specified row.
Parameters
- row
- the row to which the new cell will be added
getCellCount
public int getCellCount(int row)
Gets the number of cells on a given row.
Parameters
- row
- the row whose cells are to be counted
Return Value
the number of cells present
getFlexCellFormatter
Explicitly gets the
FlexCellFormatter. The results of
HTMLTable.getCellFormatter() may also be downcast to a
FlexCellFormatter.
Return Value
the FlexTable's cell formatter
getRowCount
public int getRowCount()
Gets the number of rows.
Return Value
number of rows
insertCell
public void insertCell(int beforeRow, int beforeColumn)
Inserts a cell into the FlexTable.
Parameters
- beforeRow
- the cell's row
- beforeColumn
- the cell's column
insertRow
public int insertRow(int beforeRow)
Inserts a row into the FlexTable.
Parameters
- beforeRow
- the row to insert
prepareCell
protected void prepareCell(int row, int column)
Ensure that the cell exists.
Parameters
- row
- the row to prepare.
- column
- the column to prepare.
prepareRow
protected void prepareRow(int row)
Ensure that the row exists.
Parameters
- row
- The row to prepare.
removeCell
public void removeCell(int row, int col)
Parameters
- row
-
- col
-
See Also
HTMLTable.removeCell(int, int)
removeCells
public void removeCells(int row, int column, int num)
Removes a number of cells from a row in the table.
Parameters
- row
- the row of the cells to be removed
- column
- the column of the first cell to be removed
- num
- the number of cells to be removed
removeRow
protected void removeRow(int row)
Removes the specified row from the table.
Parameters
- row
- the index of the row to be removed