Class HTMLTable
HTMLTable contains the common table algorithms for
Grid and
FlexTable.
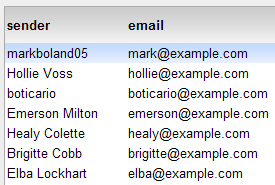
Nested Classes
Constructors
Methods
addTableListener(TableListener) | Adds a listener to the current table. |
checkCellBounds(int, int) | Bounds checks that the cell exists at the specified location. |
checkRowBounds(int) | Checks that the row is within the correct bounds. |
clear() | Removes all widgets from this table, but does not remove other HTML or text
contents of cells. |
clearCell(int, int) | Clears the given row and column. |
createCell() | Creates a new cell. |
getBodyElement() | Gets the table's TBODY element. |
getCellCount(int) | Gets the number of cells in a given row. |
getCellFormatter() | Gets the CellFormatter associated with this table. |
getCellPadding() | Gets the amount of padding that is added around all cells. |
getCellSpacing() | Gets the amount of spacing that is added around all cells. |
getColumnFormatter() | Gets the column formatter. |
getDOMCellCount(Element, int) | Directly ask the underlying DOM what the cell count on the given row is. |
getDOMCellCount(int) | Directly ask the underlying DOM what the cell count on the given row is. |
getDOMRowCount() | Directly ask the underlying DOM what the row count is. |
getDOMRowCount(Element) | |
getEventTargetCell(Event) | Determines the TD associated with the specified event. |
getHTML(int, int) | Gets the HTML contents of the specified cell. |
getRowCount() | Gets the number of rows present in this table. |
getRowFormatter() | Gets the RowFormatter associated with this table. |
getText(int, int) | Gets the text within the specified cell. |
getWidget(int, int) | Gets the widget in the specified cell. |
insertCell(int, int) | Inserts a new cell into the specified row. |
insertCells(int, int, int) | Inserts a number of cells before the specified cell. |
insertRow(int) | Inserts a new row into the table. |
internalClearCell(Element, boolean) | Does actual clearing, used by clearCell and cleanCell. |
isCellPresent(int, int) | Determines whether the specified cell exists. |
iterator() | Returns an iterator containing all the widgets in this table. |
onBrowserEvent(Event) | Method to process events generated from the browser. |
prepareCell(int, int) | Subclasses must implement this method. |
prepareColumn(int) | Subclasses can implement this method. |
prepareRow(int) | Subclasses must implement this method. |
remove(Widget) | Remove the specified widget from the table. |
removeCell(int, int) | Removes the specified cell from the table. |
removeRow(int) | Removes the specified row from the table. |
removeTableListener(TableListener) | Removes the specified table listener. |
setBorderWidth(int) | Sets the width of the table's border. |
setCellFormatter(HTMLTable.CellFormatter) | Sets the table's CellFormatter. |
setCellPadding(int) | Sets the amount of padding to be added around all cells. |
setCellSpacing(int) | Sets the amount of spacing to be added around all cells. |
setColumnFormatter(HTMLTable.ColumnFormatter) | |
setHTML(int, int, String) | Sets the HTML contents of the specified cell. |
setRowFormatter(HTMLTable.RowFormatter) | Sets the table's RowFormatter. |
setText(int, int, String) | Sets the text within the specified cell. |
setWidget(int, int, Widget) | Sets the widget within the specified cell. |
Constructor Detail
HTMLTable
public HTMLTable()
Create a new empty HTML Table.
Method Detail
addTableListener
Adds a listener to the current table.
Parameters
- listener
- listener to add
checkCellBounds
protected void checkCellBounds(int row, int column)
Bounds checks that the cell exists at the specified location.
Parameters
- row
- cell's row
- column
- cell's column
checkRowBounds
protected void checkRowBounds(int row)
Checks that the row is within the correct bounds.
Parameters
- row
- row index to check
clear
public void clear()
Removes all widgets from this table, but does not remove other HTML or text
contents of cells.
clearCell
public boolean clearCell(int row, int column)
Clears the given row and column. If it contains a Widget, it will be
removed from the table. If not, its contents will simply be cleared.
Parameters
- row
- the widget's column
- column
- the widget's column
Return Value
true if a widget was removed
createCell
Creates a new cell. Override this method if the cell should have initial
contents.
Return Value
the newly created TD
getBodyElement
Gets the table's TBODY element.
Return Value
the TBODY element
getCellCount
public abstract int getCellCount(int row)
Gets the number of cells in a given row.
Parameters
- row
- the row whose cells are to be counted
Return Value
the number of cells present in the row
getCellFormatter
Gets the
CellFormatter associated with this table. Use casting to
get subclass-specific functionality
Return Value
this table's cell formatter
getCellPadding
public int getCellPadding()
Gets the amount of padding that is added around all cells.
Return Value
the cell padding, in pixels
getCellSpacing
public int getCellSpacing()
Gets the amount of spacing that is added around all cells.
Return Value
the cell spacing, in pixels
getColumnFormatter
Gets the column formatter.
Return Value
the column formatter
getDOMCellCount
protected int
getDOMCellCount(
Element tableBody, int row)
Directly ask the underlying DOM what the cell count on the given row is.
Parameters
- tableBody
- the element
- row
- the row
Return Value
number of columns in the row
getDOMCellCount
protected int getDOMCellCount(int row)
Directly ask the underlying DOM what the cell count on the given row is.
Parameters
- row
- the row
Return Value
number of columns in the row
getDOMRowCount
protected int getDOMRowCount()
Directly ask the underlying DOM what the row count is.
Return Value
Returns the number of rows in the table
getDOMRowCount
protected int
getDOMRowCount(
Element elem)
Parameters
- elem
-
getEventTargetCell
Determines the TD associated with the specified event.
Parameters
- event
- the event to be queried
Return Value
the TD associated with the event, or
null
if none is
found.
getHTML
public
String getHTML(
int row, int column)
Gets the HTML contents of the specified cell.
Parameters
- row
- the cell's row
- column
- the cell's column
Return Value
the cell's HTML contents
getRowCount
public abstract int getRowCount()
Gets the number of rows present in this table.
Return Value
the table's row count
getRowFormatter
Gets the RowFormatter associated with this table.
Return Value
the table's row formatter
getText
public
String getText(
int row, int column)
Gets the text within the specified cell.
Parameters
- row
- the cell's row
- column
- the cell's column
Return Value
the cell's text contents
getWidget
public
Widget getWidget(
int row, int column)
Gets the widget in the specified cell.
Parameters
- row
- the cell's row
- column
- the cell's column
Return Value
the widget in the specified cell, or
null
if none is
present
insertCell
protected void insertCell(int row, int column)
Inserts a new cell into the specified row.
Parameters
- row
- the row into which the new cell will be inserted
- column
- the column before which the cell will be inserted
insertCells
protected void insertCells(int row, int column, int count)
Inserts a number of cells before the specified cell.
Parameters
- row
- the row into which the new cells will be inserted
- column
- the column before which the new cells will be inserted
- count
- number of cells to be inserted
insertRow
protected int insertRow(int beforeRow)
Inserts a new row into the table.
Parameters
- beforeRow
- the index before which the new row will be inserted
Return Value
the index of the newly-created row
internalClearCell
protected boolean
internalClearCell(
Element td, boolean clearInnerHTML)
Does actual clearing, used by clearCell and cleanCell. All HTMLTable
methods should use internalClearCell rather than clearCell, as clearCell
may be overridden in subclasses to format an empty cell.
Parameters
- td
- element to clear
- clearInnerHTML
- should the cell's inner html be cleared?
Return Value
returns whether a widget was cleared
isCellPresent
public boolean isCellPresent(int row, int column)
Determines whether the specified cell exists.
Parameters
- row
- the cell's row
- column
- the cell's column
Return Value
true
if the specified cell exists
iterator
Returns an iterator containing all the widgets in this table.
Return Value
the iterator
onBrowserEvent
public void
onBrowserEvent(
Event event)
Method to process events generated from the browser.
Parameters
- event
- the generated event
prepareCell
protected abstract void prepareCell(int row, int column)
Subclasses must implement this method. It allows them to decide what to do
just before a cell is accessed. If the cell already exists, this method
must do nothing. Otherwise, a subclass must either ensure that the cell
exists or throw an
IndexOutOfBoundsException.
Parameters
- row
- the cell's row
- column
- the cell's column
prepareColumn
protected void prepareColumn(int column)
Subclasses can implement this method. It allows them to decide what to do
just before a column is accessed. For classes, such as
FlexTable
, that do not have a concept of a global column
length can ignore this method.
Parameters
- column
- the cell's column
prepareRow
protected abstract void prepareRow(int row)
Subclasses must implement this method. If the row already exists, this
method must do nothing. Otherwise, a subclass must either ensure that the
row exists or throw an
IndexOutOfBoundsException.
Parameters
- row
- the cell's row
remove
public boolean
remove(
Widget widget)
Remove the specified widget from the table.
Parameters
- widget
- widget to remove
Return Value
was the widget removed from the table.
removeCell
protected void removeCell(int row, int column)
Removes the specified cell from the table.
Parameters
- row
- the row of the cell to remove
- column
- the column of cell to remove
removeRow
protected void removeRow(int row)
Removes the specified row from the table.
Parameters
- row
- the index of the row to be removed
removeTableListener
Removes the specified table listener.
Parameters
- listener
- listener to remove
setBorderWidth
public void setBorderWidth(int width)
Sets the width of the table's border. This border is displayed around all
cells in the table.
Parameters
- width
- the width of the border, in pixels
setCellFormatter
Sets the table's CellFormatter.
Parameters
- cellFormatter
- the table's cell formatter
setCellPadding
public void setCellPadding(int padding)
Sets the amount of padding to be added around all cells.
Parameters
- padding
- the cell padding, in pixels
setCellSpacing
public void setCellSpacing(int spacing)
Sets the amount of spacing to be added around all cells.
Parameters
- spacing
- the cell spacing, in pixels
setColumnFormatter
Parameters
- formatter
-
setHTML
public void
setHTML(
int row, int column, String html)
Sets the HTML contents of the specified cell.
Parameters
- row
- the cell's row
- column
- the cell's column
- html
- the cell's HTML contents
setRowFormatter
Sets the table's RowFormatter.
Parameters
- rowFormatter
- the table's row formatter
setText
public void
setText(
int row, int column, String text)
Sets the text within the specified cell.
Parameters
- row
- the cell's row
- column
- cell's column
- text
- the cell's text contents
setWidget
public void
setWidget(
int row, int column, Widget widget)
Sets the widget within the specified cell.
Inherited implementations may either throw IndexOutOfBounds exception if
the cell does not exist, or allocate a new cell to store the content.
FlexTable will automatically allocate the cell at the correct location and
then set the widget. Grid will set the widget if and only if the cell is
within the Grid's bounding box.
Parameters
- row
- the cell's row
- column
- the cell's column
- widget
- The widget to be added