Class Grid
A rectangular grid that can contain text, html, or a child
Widget within its cells. It must be
resized explicitly to the desired number of rows and columns.
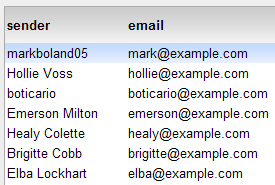
Example
public class GridExample implements EntryPoint {
public void onModuleLoad() {
// Grids must be sized explicitly, though they can be resized later.
Grid g = new Grid(5, 5);
// Put some values in the grid cells.
for (int row = 0; row < 5; ++row) {
for (int col = 0; col < 5; ++col)
g.setText(row, col, "" + row + ", " + col);
}
// Just for good measure, let's put a button in the center.
g.setWidget(2, 2, new Button("Does nothing, but could"));
// You can use the CellFormatter to affect the layout of the grid's cells.
g.getCellFormatter().setWidth(0, 2, "256px");
RootPanel.get().add(g);
}
}
Fields
numColumns | Number of columns in the current grid. |
numRows | Number of rows in the current grid. |
Constructors
Methods
Field Detail
numColumns
protected int numColumns
Number of columns in the current grid.
numRows
protected int numRows
Number of rows in the current grid.
Constructor Detail
Grid
public Grid()
Constructor for
Grid
.
Grid
public Grid(int rows, int columns)
Constructs a grid with the requested size.
Parameters
- rows
- the number of rows
- columns
- the number of columns
Method Detail
clearCell
public boolean clearCell(int row, int column)
Replaces the contents of the specified cell with a single space.
Parameters
- row
- the cell's row
- column
- the cell's column
createCell
Creates a new, empty cell.
getCellCount
public int getCellCount(int row)
Return number of columns. For grid, row argument is ignored as all grids
are rectangular.
Parameters
- row
-
getColumnCount
public int getColumnCount()
Gets the number of columns in this grid.
Return Value
the number of columns
getRowCount
public int getRowCount()
Return number of rows.
prepareCell
protected void prepareCell(int row, int column)
Checks that a cell is a valid cell in the table.
Parameters
- row
- the cell's row
- column
- the cell's column
prepareColumn
protected void prepareColumn(int column)
Checks that the column index is valid.
Parameters
- column
- The column index to be checked
prepareRow
protected void prepareRow(int row)
Checks that the row index is valid.
Parameters
- row
- The row index to be checked
resize
public void resize(int rows, int columns)
Resizes the grid.
Parameters
- rows
- the number of rows
- columns
- the number of columns
resizeColumns
public void resizeColumns(int columns)
Resizes the grid to the specified number of columns.
Parameters
- columns
- the number of columns
resizeRows
public void resizeRows(int rows)
Resizes the grid to the specified number of rows.
Parameters
- rows
- the number of rows