Class PopupPanel
A panel that can "pop up" over other widgets. It overlays the browser's
client area (and any previously-created popups).
The width and height of
the PopupPanel cannot be explicitly set; they are determined by the
PopupPanel's widget. Calls to
setWidth(String) and
setHeight(String) will call these methods on the PopupPanel's
widget.
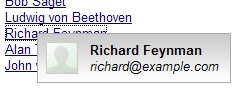
Example
public class PopupPanelExample implements EntryPoint {
private static class MyPopup extends PopupPanel {
public MyPopup() {
// PopupPanel's constructor takes 'auto-hide' as its boolean parameter.
// If this is set, the panel closes itself automatically when the user
// clicks outside of it.
super(true);
// PopupPanel is a SimplePanel, so you have to set it's widget property to
// whatever you want its contents to be.
setWidget(new Label("Click outside of this popup to close it"));
}
}
public void onModuleLoad() {
Button b1 = new Button("Click me to show popup");
b1.addClickListener(new ClickListener() {
public void onClick(Widget sender) {
// Instantiate the popup and show it.
new MyPopup().show();
}
});
RootPanel.get().add(b1);
Button b2 = new Button("Click me to show popup partway across the screen");
b2.addClickListener(new ClickListener() {
public void onClick(Widget sender) {
// Create the new popup.
final MyPopup popup = new MyPopup();
// Position the popup 1/3rd of the way down and across the screen, and
// show the popup. Since the position calculation is based on the
// offsetWidth and offsetHeight of the popup, you have to use the
// setPopupPositionAndShow(callback) method. The alternative would
// be to call show(), calculate the left and top positions, and
// call setPopupPosition(left, top). This would have the ugly side
// effect of the popup jumping from its original position to its
// new position.
popup.setPopupPositionAndShow(new PopupPanel.PositionCallback() {
public void setPosition(int offsetWidth, int offsetHeight) {
int left = (Window.getClientWidth() - offsetWidth) / 3;
int top = (Window.getClientHeight() - offsetHeight) / 3;
popup.setPopupPosition(left, top);
}
});
}
});
RootPanel.get().add(b2);
}
}
Nested Interfaces
Constructors
Methods
Constructor Detail
PopupPanel
public PopupPanel()
Creates an empty popup panel. A child widget must be added to it before it
is shown.
PopupPanel
public PopupPanel(boolean autoHide)
Creates an empty popup panel, specifying its "auto-hide" property.
Parameters
- autoHide
-
true
if the popup should be automatically
hidden when the user clicks outside of it
PopupPanel
public PopupPanel(boolean autoHide, boolean modal)
Creates an empty popup panel, specifying its "auto-hide" property.
Parameters
- autoHide
-
true
if the popup should be automatically
hidden when the user clicks outside of it
- modal
-
true
if keyboard or mouse events that do not
target the PopupPanel or its children should be ignored
Method Detail
addPopupListener
Adds a listener interface to receive popup events.
Parameters
- listener
- the listener interface to add.
center
public void center()
Centers the popup in the browser window and shows it. If the popup was
already showing, then the popup is centered.
getContainerElement
protected
Element getContainerElement()
Override this method to specify that an element other than the root element
be the container for the panel's child widget. This can be useful when you
want to create a simple panel that decorates its contents.
Return Value
the element to be used as the panel's container
getOffsetHeight
public int getOffsetHeight()
Gets the panel's offset height in pixels. Calls to
setHeight(String)
before the panel's child widget is set will not influence the offset height.
Return Value
the object's offset height
getOffsetWidth
public int getOffsetWidth()
Gets the panel's offset width in pixels. Calls to
setWidth(String)
before the panel's child widget is set will not influence the offset width.
Return Value
the object's offset width
getPopupLeft
public int getPopupLeft()
Gets the popup's left position relative to the browser's client area.
Return Value
the popup's left position
getPopupTop
public int getPopupTop()
Gets the popup's top position relative to the browser's client area.
Return Value
the popup's top position
getStyleElement
protected
Element getStyleElement()
Template method that returns the element to which style names will be
applied. By default it returns the root element, but this method may be
overridden to apply styles to a child element.
Return Value
the element to which style names will be applied
getTitle
Gets the title associated with this object. The title is the 'tool-tip'
displayed to users when they hover over the object.
Return Value
the object's title
hide
public void hide()
Hides the popup. This has no effect if it is not currently visible.
onDetach
protected void onDetach()
This method is called when a widget is detached from the browser's
document. To receive notification before the PopupPanel is removed from the
document, override the
Widget.onUnload() method instead.
onEventPreview
public boolean
onEventPreview(
Event event)
Called when a browser event occurs and this event preview is on top of the
preview stack.
Parameters
- event
- the browser event
Return Value
false
to cancel the event
See Also
DOM.addEventPreview(EventPreview)
onKeyDownPreview
public boolean onKeyDownPreview(char key, int modifiers)
Popups get an opportunity to preview keyboard events before they are passed
to a widget contained by the Popup.
Parameters
- key
- the key code of the depressed key
- modifiers
- keyboard modifiers, as specified in
KeyboardListener.
Return Value
false
to suppress the event
onKeyPressPreview
public boolean onKeyPressPreview(char key, int modifiers)
Popups get an opportunity to preview keyboard events before they are passed
to a widget contained by the Popup.
Parameters
- key
- the unicode character pressed
- modifiers
- keyboard modifiers, as specified in
KeyboardListener.
Return Value
false
to suppress the event
onKeyUpPreview
public boolean onKeyUpPreview(char key, int modifiers)
Popups get an opportunity to preview keyboard events before they are passed
to a widget contained by the Popup.
Parameters
- key
- the key code of the released key
- modifiers
- keyboard modifiers, as specified in
KeyboardListener.
Return Value
false
to suppress the event
removePopupListener
Removes a previously added popup listener.
Parameters
- listener
- the listener interface to remove.
setHeight
public void
setHeight(
String height)
Sets the height of the panel's child widget. If the panel's child widget
has not been set, the height passed in will be cached and used to set
the height immediately after the child widget is set.
Parameters
- height
- the object's new height, in CSS units (e.g. "10px", "1em")
setPopupPosition
public void setPopupPosition(int left, int top)
Sets the popup's position relative to the browser's client area. The
popup's position may be set before calling
show().
Parameters
- left
- the left position, in pixels
- top
- the top position, in pixels
setPopupPositionAndShow
Sets the popup's position using a
PositionCallback, and shows
the popup. The callback allows positioning to be performed based on
the offsetWidth and offsetHeight of the popup, which are normally
not available until the popup is showing. By positioning the popup
before it is shown, the the popup will not jump from its original
position to the new position.
Parameters
- callback
- the callback to set the position of the popup
See Also
PositionCallback.setPosition(int offsetWidth, int offsetHeight)
setTitle
public void
setTitle(
String title)
Sets the title associated with this object. The title is the 'tool-tip'
displayed to users when they hover over the object.
Parameters
- title
- the object's new title
setVisible
public void setVisible(boolean visible)
Sets whether this object is visible.
Parameters
- visible
-
true
to show the object, false
to hide it
setWidget
public void
setWidget(
Widget w)
Sets this panel's widget. Any existing child widget will be removed.
Parameters
- w
- the panel's new widget, or
null
to clear the panel
setWidth
public void
setWidth(
String width)
Sets the width of the panel's child widget. If the panel's child widget
has not been set, the width passed in will be cached and used to set
the width immediately after the child widget is set.
Parameters
- width
- the object's new width, in CSS units (e.g. "10px", "1em")
show
public void show()
Shows the popup. It must have a child widget before this method is called.